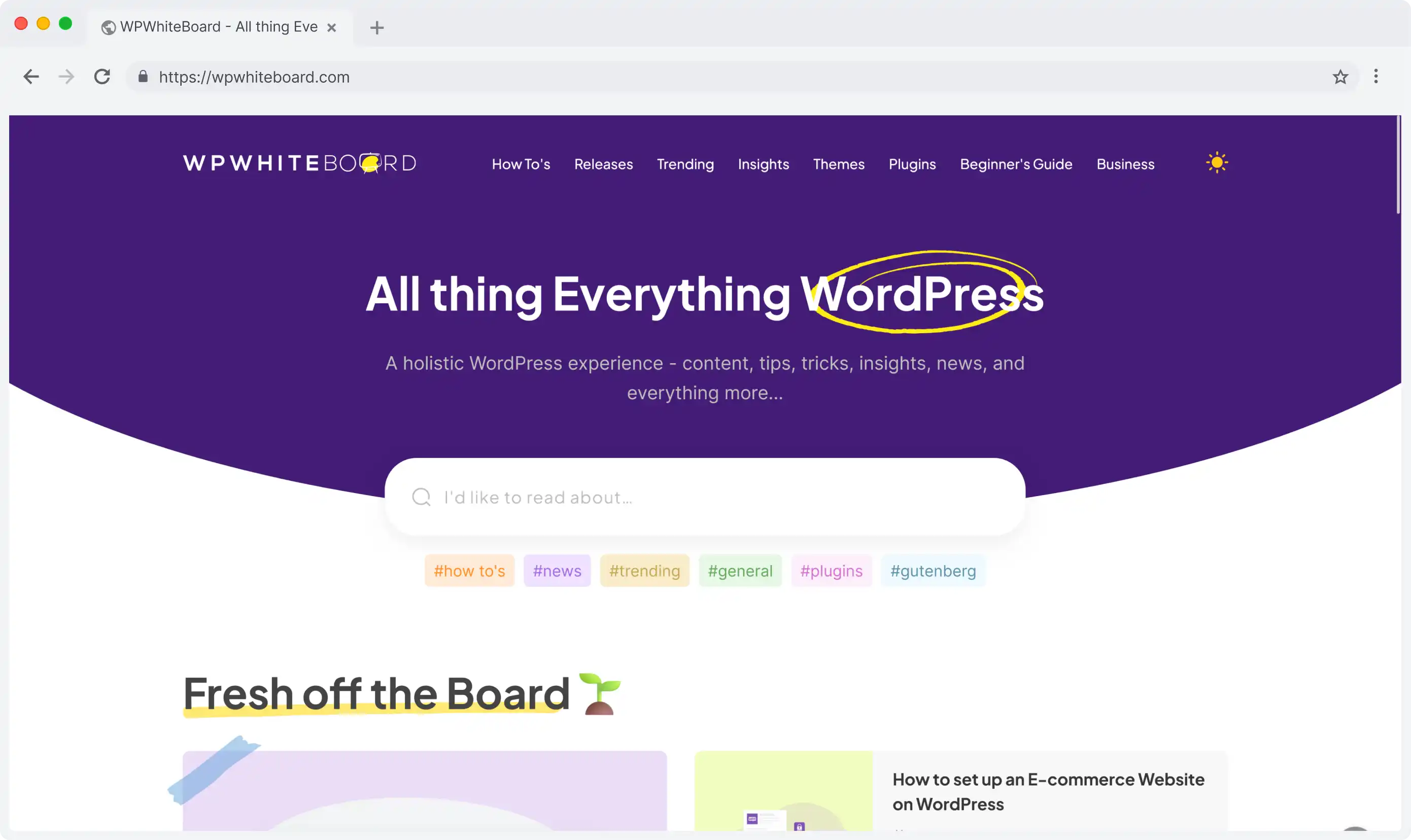
Headless CMS scales and improves WPWhiteBoard’s content distribution, flexibility, and personalization
Retail & E-commerce
Analyze conversion rates and improve sales. Drive eCom Experience to Success.
Healthcare
Deliver best-in-class care experience. Drive digital to supercharge patient care.
Technology
Turnkey solutions to maximize your revenue. Retention drivers to amplify growth.
Manufacturing
The best of efficiency and effectiveness to maximize output. Drive best results.
Headless CMS scales and improves WPWhiteBoard’s content distribution, flexibility, and personalization
Driving Cutting-Edge AI Innovation in Document Intelligence Through Targeted Cloud Optimization
Empowering a Seamless Content Management Transition for Humans of Globe