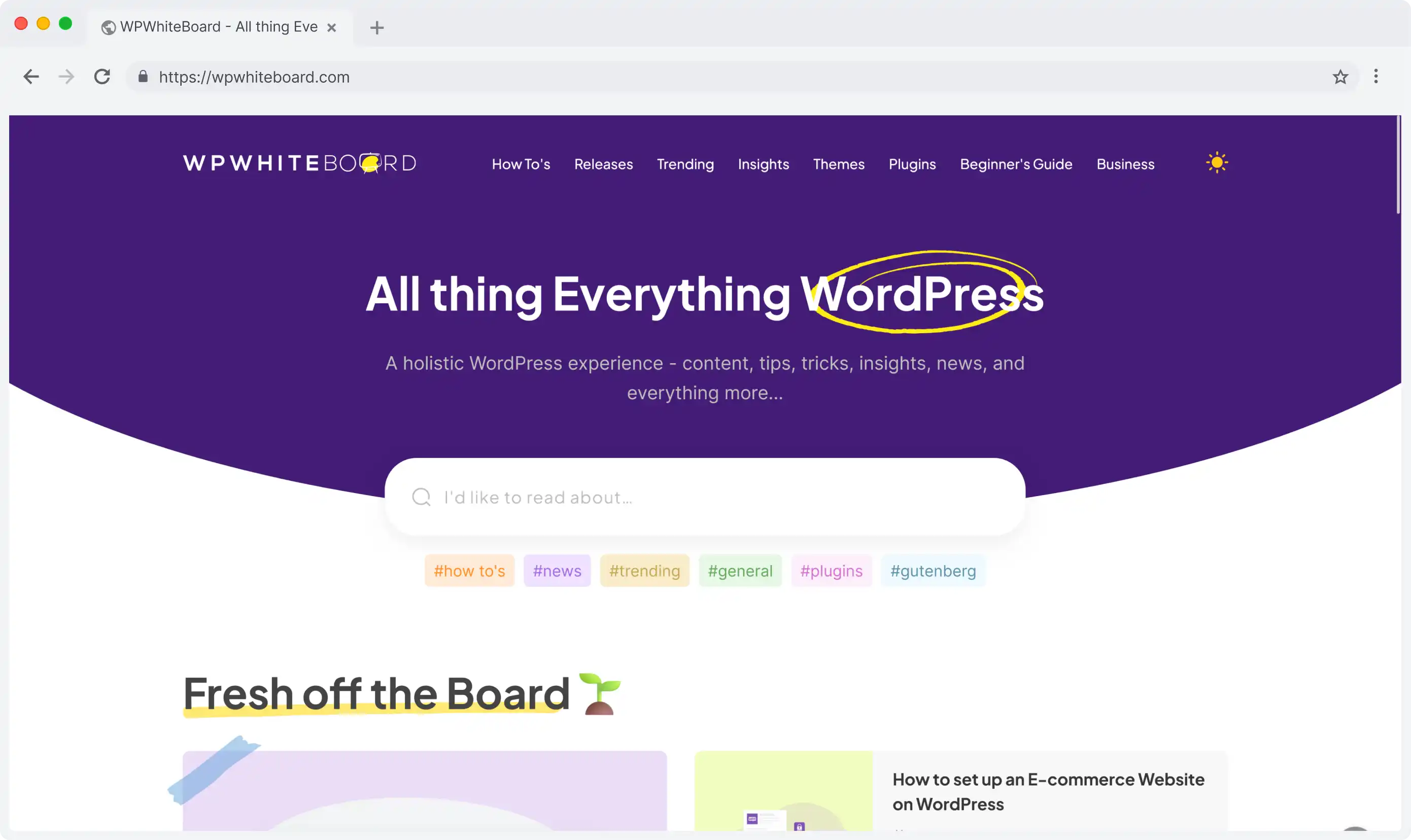
Headless CMS scales and improves WPWhiteBoard’s content distribution, flexibility, and personalization
Retail & E-commerce
Analyze conversion rates and improve sales. Drive eCom Experience to Success.
Healthcare
Deliver best-in-class care experience. Drive digital to supercharge patient care.
Technology
Turnkey solutions to maximize your revenue. Retention drivers to amplify growth.
Manufacturing
The best of efficiency and effectiveness to maximize output. Drive best results.
Headless CMS scales and improves WPWhiteBoard’s content distribution, flexibility, and personalization
Driving Cutting-Edge AI Innovation in Document Intelligence Through Targeted Cloud Optimization
Empowering a Seamless Content Management Transition for Humans of Globe
~300%
Increase in monthly website visits
25%
Boost in referral traffic
3X
Faster page load speeds
We saw immense gains in site traffic, user engagement, and performance after adopting the consultants’ headless approach.
Chief Editor
60%
Cost reduction in infrastructure
4X
Improvement in page load speeds
99.95%
Uptime across product portfolio
The headless platform has been instrumental in nurturing relentless experimentation with new capabilities. Our rapid growth is a testament to...
CTO
61%
Boost in mobile page load speeds
236%
Rise in organic traffic
23%
Rise in membership conversion rates
WPSteroids enabled us to incrementally refine our architecture to balance practicality, costs, and future-readiness while fueling innovation
CEO